SECRET
DigiMastered Works LLC
Office of Development
DMW107IB7002
Date 09/03/2021 02:25PM
To: The People
From: Tanner Fry
Subject: Finding a Way
UI Implementation
Over the last two months the UI has been completely changed from an arcade-like style to a more digital/clean look. This can be seen later via the Feedback UI but more will be shown in due time. Finding a proper theme for the UI took longer than expected. It never felt right until a balance was found that brought about the order of what intelligence agencies have and the the digital feel of the era. The game takes place in 2054 where the world has been ravaged by the nature of humans. With destruction at every turn, some civilizations have been able to rebuild their country anew.
This setting is what fueled the change from an arcade-like style to a more digital/clean look.
Major Changes
Database Management
To make a fluent game, it needs the flow of data at any time. In the act of achieving this flow, many look towards relational or non relational databases. Though I wondered which I might take, the few database management classes I took in college seemed to give enough knowledge to build what was needed. Creating tables and linking the database to the game for CRUD operations is trivial for experienced developers but if you're not one, then below an example will be given for how I've done so.
using Mono.Data.Sqlite;
using System.Data;
using UnityEngine;
public class DatabaseMananger : MonoBehaviour {
string connection;
string sqlQuery;
IDbConnection dbConnection;
IDbCommand dbCommand;
string DATABASE_NAME = "/Databases/aia-database.db";
void Start() {
// Create the connection to the database
string filepath = Application.dataPath + DATABASE_NAME;
connection = "Data Source= " + filepath + ";Version=3;";
dbConnection = new SqliteConnection(connection);
// Creating director table with basic fields
private void CreateDirectorTable() {
using (dbConnection = new SqliteConnection(connection)) {
dbConnection.Open();
dbCommand = dbConnection.CreateCommand();
sqlQuery = "CREATE TABLE IF NOT EXISTS DirectorTable (" +
"[id] INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT," +
"[save_id] INTEGER NOT NULL," +
"[name] VARCHAR(255) NOT NULL," +
"[title] VARCHAR(255) DEFAULT 'Director' NOT NULL," +
"[age] INTEGER DEFAULT '18' NOT NULL)";
dbCommand.CommandText = sqlQuery;
dbCommand.ExecuteScalar();
dbConnection.Close();
}
}
// Inserting into DirectorTable
private void TestInsertDirectorTable(int save_id, string name, string title,
int age)
{
using (dbConnection = new SqliteConnection(connection)) {
dbConnection.Open();
dbCommand = dbConnection.CreateCommand();
sqlQuery = "INSERT INTO DirectorTable VALUES (NULL, " +
save_id + ", '" +
name + "', '" +
title + "', " +
age + ")";
dbCommand.CommandText = sqlQuery;
dbCommand.ExecuteScalar();
dbConnection.Close();
}
Debug.Log("Database table DirectorTable had a new record inserted.");
}
// Selecting from DirectorTable
public string SelectDirectorFromDirectorTable(int saveID, string name, string title,
int age)
{
string directorInfo = "";
using (dbConnection = new SqliteConnection(connection)) {
dbConnection.Open();
dbCommand = dbConnection.CreateCommand();
// This should be modifyable depending on what needs to be gathered
sqlQuery = "SELECT * FROM DirectorTable WHERE save_id = '" + saveID + "'";
dbCommand.CommandText = sqlQuery;
IDataReader responseReader = dbCommand.ExecuteReader();
while (responseReader.Read()) {
directorInfo = responseReader.GetInt32(1) + " " +
responseReader.GetString(2) + " " +
responseReader.GetString(3) + " " + responseReader.GetInt32(4);
Debug.Log("Database table DirectorTable returned: " + directorInfo);
}
responseReader.Close();
dbConnection.Close();
}
return directorInfo;
}
}
NOTES:
Gathering Feedback and Issues With Reporting
When developers create games, one of the most important parts of the process is gathering feedback from the players. I've applied a user interface to do this and even implemented a way for emails to be sent to support@digimasteredworks.com.
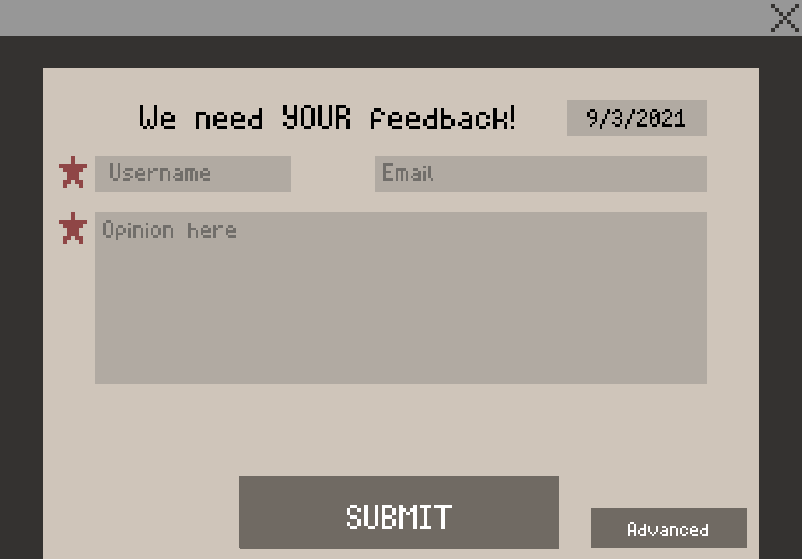
Feedback UI for players to submit their opinions.
However, when building this solution a massive security flaw started to become apparent. The issue was that credentials had to be hard coded into the software. The way it was developed was to use the support email to send the feedback to itself with the players input in the email. A solution to hard coding it was to hash the credentials into the database but sending the credentials to the Outlook server was still needed. Therefore, the credentials still needed to be sent in plaintext at some point in time (Plaintext could still be encrypted over the internet).
Solution? Send a HTML POST to digimasteredworks.com/rest-api/aia/feedback where a script would handle the data. This hasn't been done yet, but seems to be the best solution to the issue. Some concerns would be DDOS attacks which could bring down the site, creating the script to take in POST data, as well as inserting the data into a database.
Use of Prefabs
On my journey through the creation of The Greatest Cold War, I realized the importance of prefabs. To preface, AIA is being built using the Unity game engine. Prefabs act as templates for GameObjects in Unity. They can store all of the GameObject's components, some property values, and child GameObjects for reusability. Through my early ignorance, I avoided prefabs like the plague. With no understanding of them, there was a fear to learn and thus it held me back.
Lessons Learned and Issues
Through the last few months of development, many lessons have come to light. The use of prefabs, data management, the importance of gathering feedback for improvements, the misuse of commas, and the need to handle POST data.
Some issues that will need to be addressed in the future are the changing of the game's resolution and correct creation of database tables with the right foreign keys to allow the necessary information to be gathered.
I hoped you learned something new today and if you have any questions, feel free to reach out!
Roadmap
Milestones To Do:
Milestones Completed:
SECRET
Approved for release: 09/03/2021 ID: IB7002
1